Tips for Implementing Server-Side Rendering (SSR) on Your Dedicated Server
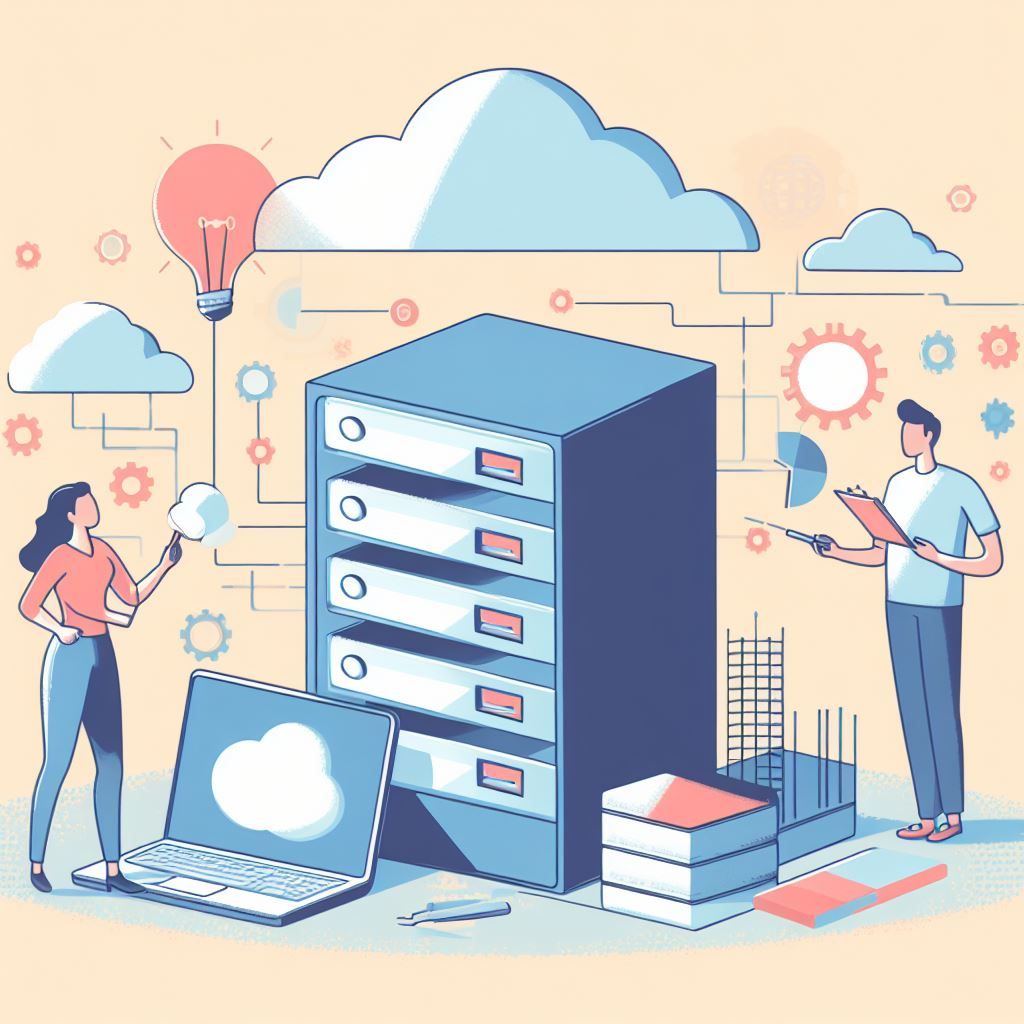
Implementing Server-Side Rendering (SSR) on your dedicated server is a powerful technique to improve the performance and SEO of your web application. Here are some tips to help you get started:
- Choose a Framework or Library: Use a JavaScript framework or library that supports SSR. Popular options include Next.js for React applications, Nuxt.js for Vue.js applications, and Angular Universal for Angular applications.
- Set Up Node.js: Ensure that Node.js is installed on your dedicated server. SSR relies on server-side JavaScript execution, and Node.js is the most common environment for this purpose.
- Install Required Dependencies: Install the necessary packages for SSR. For example, if you're using Next.js, you'll need to install
next
and related dependencies. - Configure Your Web Server: If you're using a web server like NGINX or Apache, you'll need to configure it to handle SSR requests. This typically involves setting up reverse proxies to forward requests to your Node.js application.
- Optimize for SEO: SSR inherently improves SEO since search engines can crawl and index the server-rendered content. Ensure that your application generates proper metadata (title, description, etc.) on the server side.
- Handle Routing: Configure server-side routing. This ensures that your application can handle different routes and serve the appropriate content for each one.
- Manage State and Data Fetching: If your application relies on fetching data, consider how you'll handle this on the server side. You might need to fetch data before rendering the page and pass it as props to your components.
- Client-Side Hydration: After the initial server-rendered content is delivered to the client, the client-side JavaScript takes over. Ensure that client-side hydration is set up correctly so that the application becomes interactive.
- Optimize for Performance: Even with SSR, you should still focus on performance. This includes optimizing images, using lazy loading, and minimizing unnecessary JavaScript.
- Use Caching: Implement caching strategies to reduce server load and improve response times. You can use techniques like memoization or server-level caching mechanisms.
- Handle Authentication and Authorization: If your application requires user authentication, ensure that this is handled on both the server and client sides. This might involve passing authentication tokens to the client.
- Set Up Error Handling: Implement proper error handling to gracefully deal with server errors and client-side errors.
- Monitor Server Performance: Use monitoring tools to keep an eye on the performance of your dedicated server. This includes CPU and memory usage, response times, and error rates.
- Security Considerations: Always follow best practices for server security. This includes setting up firewalls, keeping software up to date, and following security guidelines for your chosen framework or library.
- Testing: Rigorously test your application, especially in SSR scenarios. This includes testing server-rendered components, routing, and data fetching.
- Logging and Debugging: Implement robust logging and debugging mechanisms. This helps in identifying and troubleshooting issues in production.
- Scaling Considerations: As your application grows, you may need to think about scaling strategies such as load balancing, horizontal scaling, and caching layers.
Remember to regularly update your dependencies and stay informed about best practices in SSR and server-side development to ensure your application remains secure and performs optimally.