How to Implement Microservices Architecture on Your Dedicated Server
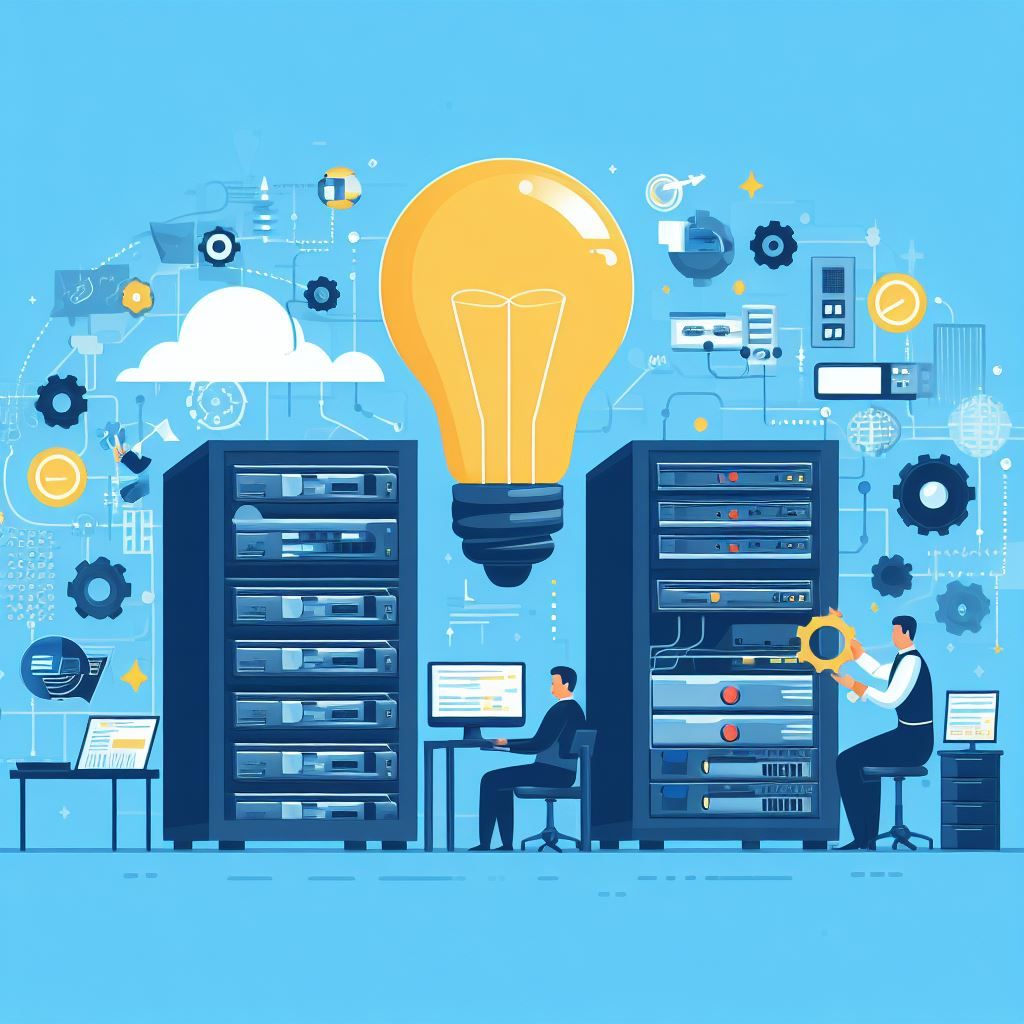
Implementing a microservices architecture on a dedicated server involves breaking down a large application into smaller, loosely coupled services that can be developed, deployed, and maintained independently. Here's a step-by-step guide to help you get started:
- Select a Programming Language and Framework:
- Choose a language and framework that best suits your project. Common choices include Java/Spring Boot, Python/Django, Node.js/Express, Go, etc.
- Define Service Boundaries:
- Identify the components of your application that can be isolated as separate services. Each service should be responsible for a specific business capability.
- Set Up Version Control:
- Use a version control system like Git to manage your codebase. Create repositories for each microservice.
- Database Management:
- Decide whether each service will have its own database or if they'll share a common database. Consider tools like PostgreSQL, MySQL, or NoSQL databases depending on your requirements.
- Service-to-Service Communication:
- Decide on a method for services to communicate with each other. This could be synchronous (HTTP/REST, GraphQL) or asynchronous (message queues, event-driven architectures).
- Containerization:
- Use containers (e.g., Docker) to package each microservice along with its dependencies. This ensures consistency across different environments.
- Orchestration:
- Consider using container orchestration tools like Kubernetes to manage and scale your containers.
- API Gateway (Optional):
- Implement an API gateway to act as a single entry point for external clients. It can handle routing, authentication, rate limiting, etc.
- Service Discovery and Load Balancing:
- Set up tools like Consul or Kubernetes' built-in service discovery to help services locate and communicate with each other.
- Configuration Management:
- Use tools like environment variables, configuration files, or a dedicated service like Consul or Zookeeper to manage configuration across services.
- Logging and Monitoring:
- Implement logging and monitoring solutions to track the health and performance of your microservices. Tools like Prometheus, Grafana, ELK Stack, or similar can be used.
- Security and Authorization:
- Implement authentication and authorization mechanisms to ensure that only authorized services can communicate with each other. Use techniques like OAuth, JWT, or API keys.
- Testing:
- Write unit tests, integration tests, and end-to-end tests for each microservice. Implement continuous integration and continuous deployment (CI/CD) pipelines.
- Deployment and Scaling:
- Use deployment tools like Kubernetes, Docker Swarm, or other container orchestration platforms for deploying and scaling your microservices.
- Error Handling and Resilience:
- Implement mechanisms for graceful degradation and fallback strategies to handle errors and failures gracefully.
- Documentation:
- Maintain thorough documentation for each microservice, including API documentation, dependencies, and deployment instructions.
- Continuous Monitoring and Optimization:
- Monitor the performance and resource utilization of your microservices and make adjustments as necessary. Optimize for efficiency and cost-effectiveness.
- Backup and Disaster Recovery:
- Implement regular backups and have a disaster recovery plan in place in case of any unforeseen incidents.
Remember that implementing microservices is a complex task, and the specifics can vary greatly depending on your application, technology stack, and business requirements. It's crucial to thoroughly plan, document, and test your architecture to ensure its success.